(2022/03/09 更新)
Listはデータの一覧表示をするのに適したViewです。
画面に収まらない量の場合はスクロール表示になるなど、UIKitのUITableViewに似ていますが、はるかに簡単に使えます。
ListはForEachとセットで使うケースが多いので、先にこちらを読んで理解しておくことをおすすめします。
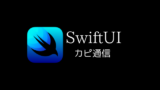
環境
この記事の情報は次のバージョンで動作確認しています。
【Swift】5.5.2
【iOS】15.2
【macOS】Monterey バージョン 12.2.1
静的リストの生成
一覧に表示したいViewをListに並べるだけで静的リストが生成できます。
1 2 3 4 5 6 7 8 9 10 11 |
struct ContentView: View { var body: some View { List { Text("大根") Text("キャベツ") Text("じゃがいも") } } } |
動的リストの生成
ForEachを使うと配列などから動的に一覧を生成できます。
1 2 3 4 5 6 7 8 9 10 11 12 |
struct ContentView: View { let fruits = ["りんご", "オレンジ", "バナナ"] var body: some View { List { ForEach(0 ..< fruits.count) { index in Text(fruits[index]) } } } } |
静的リストと動的リストの混在
静的行と動的行の混在も可能です。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
struct ContentView: View { let fruits = ["りんご", "オレンジ", "バナナ"] var body: some View { List { Text("大根") Text("キャベツ") Text("じゃがいも") ForEach(0 ..< fruits.count) { index in Text(fruits[index]) } } } } |
リストのグループ化
Formと同じ様にSectionと組み合わせて、リストをグループ化できます。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
struct ContentView: View { let fruits = ["りんご", "オレンジ", "バナナ"] var body: some View { List { Section { Text("大根") Text("キャベツ") Text("じゃがいも") } header: { Text("やさい") } Section { ForEach(0 ..< fruits.count) { index in Text(fruits[index]) } } header: { Text("くだもの") } } } } |
シンプルな動的リスト
単一ループによる動的リストであれば、ForEachを使わずにListのみでも生成できます。
使い方はForEachと同じで、「範囲指定」と「データ指定」のどちらも可能。
行の削除や入れ替えには対応できない為、使える範囲は限定されます。
単純な動的リストを出力する為のシンプルな表記方法です。
1 2 3 4 5 6 7 8 9 10 |
struct ContentView: View { let fruits = ["りんご", "オレンジ", "バナナ"] var body: some View { List(fruits, id: \.self) { fruit in Text(fruit) } } } |
表示スタイルの指定
1 2 3 |
.listStyle(スタイル指定) |
listStyle()モディファイアを使ってListの表示スタイルを指定可能です。
未指定の場合は、.listStyle(DefaultListStyle()) となり、状況に応じて自動でスタイルが選択されます。
選択されるスタイルはOSのバージョンによっても変わる場合がある為、アプリのデザインを固定したい場合には明示的にスタイルを指定する必要があります。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
struct ContentView: View { let fruits = ["りんご", "オレンジ", "バナナ"] var body: some View { List { Section { Text("大根") Text("キャベツ") Text("じゃがいも") } header: { Text("やさい") } Section { ForEach(0 ..< fruits.count) { index in Text(fruits[index]) } } header: { Text("くだもの") } } .listStyle(DefaultListStyle()) // Listの表示スタイル指定 } } |
PlainListStyle()
1 2 3 4 5 6 7 |
.listStyle(PlainListStyle()) または .listStyle(.plain) |
特別な装飾なしのプレーン表示スタイル。
GroupedListStyle()
1 2 3 4 5 6 7 |
.listStyle(GroupedListStyle()) または .listStyle(.grouped) |
グループ化されたリストの表示スタイル。
InsetListStyle()
1 2 3 4 5 6 7 |
.listStyle(InsetListStyle()) または .listStyle(.inset) |
はめ込みリストの表示スタイル。
リストの周りにマージンが適用される。
iOS14以降で使用可能。
InsetGroupedListStyle()
1 2 3 4 5 6 7 |
.listStyle(InsetGroupedListStyle()) または .listStyle(.insetGrouped) |
はめ込み+グループ化リストの表示スタイル。
iOS14以降で使用可能。
SidebarListStyle()
1 2 3 4 5 6 7 |
.listStyle(SidebarListStyle()) または .listStyle(.sidebar) |
サイドバー用のスタイル。
リストを折りたためる表示スタイル。
iOS14以降で使用可能。
リストの最後に空白を挿入する
Spacer()を使ってリストの最後に空白を挿入可能です。
引数minLengthで空白のサイズを指定します。
サイズ指定がない場合は1行分のみ挿入されます。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
struct ContentView: View { var body: some View { List { ForEach(1 ..< 101) { index in Text("\(index)行目") } /// リストの最後に空白を入れる Spacer(minLength: 200) } } } |
あわせて読みたい記事
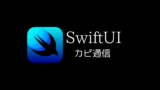
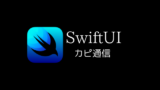
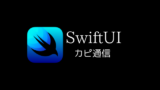
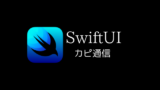
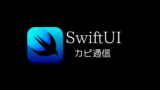