(2021/08/24 更新)
Swiftでの文字列の扱い方をまとめました。
環境
この記事の情報は次のバージョンで動作確認しています。
【Swift】5.4
【iOS】14.5
【macOS】Big Sur バージョン 11.5.2
結合
文字列の結合には演算子(+)および代入演算子(+=)を使います。
1 2 3 4 5 6 7 8 9 10 |
var word1 = "I Love " let word2 = "Swift" let message = word1 + word2 print(message) // I love Swift word1 += "Apple" print(word1) // I love Apple |
比較
数値の比較と同様の比較演算子(==, !=, <, >)が使えます。
1 2 3 4 5 6 7 8 9 10 11 12 |
var word1 = "Swift" var word2 = "SwiftUI" if word1 == word2 { print("2つの文字列は等しい") } else { print("2つの文字列は等しくない") } // "2つの文字列は等しくない" |
文字列→数値変換
文字列から数値への変換にはInt()やDouble()を使います。
変換できない場合はnilになります。
1 2 3 4 5 6 7 8 |
let strNum = "123" let intNum = Int(strNum) let doubleNum = Double(strNum) print(intNum) // Optional(123) print(doubleNum) // Optional(123.0) |
数値→文字列変換
数値から文字列への変換には、String()を使います。
1 2 3 4 5 6 7 |
let intNum = 123 let doubleNum = 123.4 print(String(intNum)) // "123" print(String(doubleNum)) // "123.4" |
\()を使ってプロパティの値を文字列に埋め込みむ方法もあります。
("\"はキーボードのoption+"¥"で入力します。)
1 2 3 4 5 6 7 8 |
let intNum = 123 let doubleNum = 123.4 let string = "\(intNum) \(doubleNum)" print(string) // "123 123.4" |
文字列で使える特殊文字(エスケープシーケンス)
("\"はキーボードのoption+"¥"で入力します。)
\" | ダブルクォート |
\' | シングルクォート |
\n | 改行 |
\t | タブ |
\\ | バックスラッシュ |
文字数を返す
countプロパティで文字数を取得できます。
1 2 3 4 5 6 7 |
let message1 = "I love Swift" let message2 = "カピ通信" print(message1.count) // 12 print(message2.count) // 4 |
大文字/小文字変換
文字列の大文字/小文字変換は、uppercased()とlowercased()を使用します。
1 2 3 4 5 6 |
let message = "I love Swift." print(message.uppercased()) // "I LOVE SWIFT." print(message.lowercased()) // "i love swift." |
前後の空白を削除する
trimmingCharacters(in:)は、文字列の両端から指定された文字セットを削除した新たな文字列を返します。
引数に文字セット.whitespacesAndNewlinesを指定すると、両端にあるスペース、タブ、改行を全て削除した文字列を返します。
1 2 3 4 5 6 |
let title = " カピ通信 \t \n" let trimedTitle = title.trimmingCharacters(in: .whitespacesAndNewlines) print(trimedTitle) // "カピ通信" |
空文字かどうかチェックする
isEmptyプロパティで空文字かどうかチェックできます。
空文字の場合はtrueを返します。
1 2 3 4 5 6 7 8 9 10 |
let title = "カピ通信" let title2 = "" let title3 = " " print(title.isEmpty) // false print(title2.isEmpty) // true print(title3.isEmpty) // false print(title3.trimmingCharacters(in: .whitespacesAndNewlines).isEmpty) // true |
分割
components(separatedBy:)で文字列を区切り文字で分割した配列にします。
1 2 3 4 5 6 |
let wordList = "apple swift xcode" let words = wordList.components(separatedBy: " ") print(words) // ["apple", "swift", "xcode"] |
文字列を1文字づつ扱う
for-inループを使用して、配列のように全ての文字にアクセス可能です。
1 2 3 4 5 6 |
let word = "Apple" for letter in word { print(letter) } |
出力
1 2 3 4 5 6 7 |
A p p l e |
文字列を配列に変換する
Array()で1文字つづの配列に変換できます。
1 2 3 4 |
let letter = Array("Apple") print(letter) // ["A", "p", "p", "l", "e"] |
ランダムな要素を返す
randomElement()で、文字列からランダムに文字を返します。
1 2 3 4 5 6 7 |
let youbi = "日月火水木金土" print(youbi.randomElement()) // Optional("火") print(youbi.randomElement()) // Optional("土") print(youbi.randomElement()) // Optional("月") |
最初に見つかった特定文字を削除する
firstIndex(of:)で、指定した文字が最初に見つかった文字列中の位置(String.Index型)を取得します。
次に、remove(at:)に取得した位置情報を渡して該当文字を削除します。
位置情報はハッシュ値で保持されている為、直接何文字目か指定はできません。
1 2 3 4 5 6 7 8 9 |
/// 文字列中の","を削除する var date = "April 24, 2020" if let pos = date.firstIndex(of: ",") { date.remove(at: pos) } print(date) // April 24 2020 |
文字列の一部を取り出す
swiftのStringには一般的なsubstring関数がありません。
文字列の一部を取り出すには、若干工夫が必要です。
任意の位置からの取り出し
index()関数を使って、開始位置と終了位置を、先頭からの相対位置で取得します。
相対位置は先頭が0になりますので、3文字目なら2、7文字目なら6になります。
開始位置と終了位置を使って、取得する文字列の範囲を指定しますが、
ここで取得した文字列は、Substring型になる為、最後にString型にキャストします。
以下は、3文字目から7文字目までを取り出す例です。
1 2 3 4 5 6 7 8 9 |
let word: String = "1234567890" let start = word.index(word.startIndex, offsetBy: 2) // 開始位置 let end = word.index(word.startIndex, offsetBy: 6) // 終了位置 let newWord = String(word[start...end]) // 文字列取得 print(newWord) // "34567" |
先頭または末尾から取り出し
先頭から指定した文字数を取り出すのは、次のようにprefix()関数で取り出す文字数を指定するだけです。
先程と同様に最後にString型にキャストする必要があります。
1 2 3 4 5 6 7 |
let word: String = "1234567890" let newWord = String(word.prefix(5)) print(newWord) // "12345" |
末尾からの取り出しは、suffix()関数を指定します。
1 2 3 4 5 6 7 |
let word: String = "1234567890" let newWord = String(word.suffix(5)) print(newWord) // "67890" |
あわせて読みたい記事
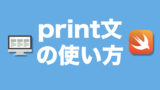
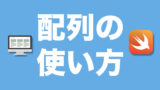